Category | Difficulty | Likes | Dislikes |
---|
algorithms | Medium (71.30%) | 1773 | - |
Tags
linked-list
Companies
bloomberg
| microsoft
| uber
给你一个链表,两两交换其中相邻的节点,并返回交换后链表的头节点。你必须在不修改节点内部的值的情况下完成本题(即,只能进行节点交换)。
示例 1:
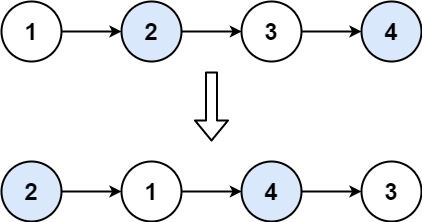
1
2
| 输入:head = [1,2,3,4]
输出:[2,1,4,3]
|
示例 2:
示例 3:
提示:
- 链表中节点的数目在范围
[0, 100]
内 0 <= Node.val <= 100
Discussion | Solution
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
| // @lc code=start
// Definition for singly-linked list.
// #[derive(PartialEq, Eq, Clone, Debug)]
// pub struct ListNode {
// pub val: i32,
// pub next: Option<Box<ListNode>>
// }
//
// impl ListNode {
// #[inline]
// fn new(val: i32) -> Self {
// ListNode {
// next: None,
// val
// }
// }
// }
impl Solution {
/// ## 解题思路
/// - 指针交换
pub fn swap_pairs(head: Option<Box<ListNode>>) -> Option<Box<ListNode>> {
let mut dummy = Box::new(ListNode { val: 0, next: head }); //add dummy_head before head
let mut p_ref = dummy.as_mut();
while p_ref.next.is_some() && p_ref.next.as_ref().unwrap().next.is_some() {
if let Some(mut first) = p_ref.next.take() {
if let Some(mut second) = first.next.take() {
first.next = second.next.take();
second.next = Some(first);
p_ref.next = Some(second);
p_ref = p_ref.next.as_mut().unwrap();
p_ref = p_ref.next.as_mut().unwrap();
}
}
}
dummy.next
}
}
// @lc code=end
|