Category | Difficulty | Likes | Dislikes |
---|
algorithms | Easy (49.24%) | 656 | - |
Tags
tree
| depth-first-search
| breadth-first-search
Companies
Unknown
给定一个二叉树,找出其最小深度。
最小深度是从根节点到最近叶子节点的最短路径上的节点数量。
说明:叶子节点是指没有子节点的节点。
示例 1:
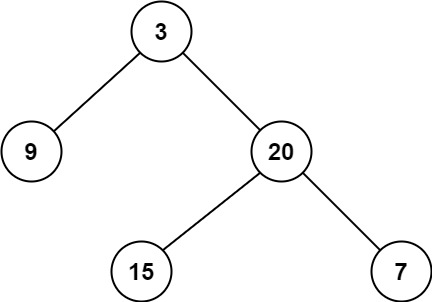
1
2
| 输入:root = [3,9,20,null,null,15,7]
输出:2
|
示例 2:
1
2
| 输入:root = [2,null,3,null,4,null,5,null,6]
输出:5
|
提示:
- 树中节点数的范围在
[0, 105]
内 -1000 <= Node.val <= 1000
Discussion | Solution
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
| // @lc code=start
// Definition for a binary tree node.
// #[derive(Debug, PartialEq, Eq)]
// pub struct TreeNode {
// pub val: i32,
// pub left: Option<Rc<RefCell<TreeNode>>>,
// pub right: Option<Rc<RefCell<TreeNode>>>,
// }
//
// impl TreeNode {
// #[inline]
// pub fn new(val: i32) -> Self {
// TreeNode {
// val,
// left: None,
// right: None
// }
// }
// }
use std::cell::RefCell;
use std::rc::Rc;
impl Solution {
/// ## 解题思路
/// - 递归
pub fn min_depth(root: Option<Rc<RefCell<TreeNode>>>) -> i32 {
match &root {
None => 0,
Some(node) => {
let left_depth = Self::min_depth(node.borrow().left.clone());
let right_depth = Self::min_depth(node.borrow().right.clone());
if left_depth > 0 && right_depth > 0 {
1 + left_depth.min(right_depth)
} else {
1 + left_depth.max(right_depth)
}
}
}
}
}
// @lc code=end
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
| class Solution {
public:
/*
## 解题思路
* 递归法:
*
*/
int minDepth(TreeNode* root) {
if (!root) return 0;
int l = minDepth(root->left) ;
int r = minDepth(root->right) ;
return 1 + (l&&r ? min(l,r) : max(l, r));
}
};
|